일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
- programming
- Class
- 알고리즘
- predictive analysis
- Deep Learning
- Data Science
- 티스토리챌린지
- baekjoon
- pointer
- array
- 백준
- function
- OOP
- assignment operator
- C++
- Pre-processing
- vscode
- 오블완
- 포인터
- 문자열
- Object Oriented Programming
- 배열
- 반복문
- const
- 함수
- pass by reference
- raw data
- string
- Python
- 파이썬
- Today
- Total
Channi Studies
[Sorting] Introduction & Selection Sort 본문
[Sorting] Introduction & Selection Sort
Chan Lee 2025. 3. 16. 07:09Sorting
Sorting is the process of converting a list of elements into ascending (or descending) order.
You may remember the importance of sorting in binary search from my previous post.
In the following few posts, we will be studying about the types of sorting algorithms.
These are some examples of (un)sorted data
(3, 9, 44, 18, 76): Not sorted
(20, 15, 10, 5, 0): Sorted into descending order
(99.87, 99.01, 67.30, 44.51): Sorted into descending order
('F', 'D', 'C', 'B', 'A',): Sorted into descending order
('chopstick', 'fork', 'knife', 'spoon'): Sorted into ascending order
('great', 'greater', 'greatest'): Sorted into ascending order
Selection Sort
Selection sort is a sorting algorithm that treats the input as two parts, a sorted part and an unsorted part, and repeatedly selects the proper next value to move from the unsorted part to the end of the sorted part.
We will go through each index (i) starting from 0 to len(arr) - 1 and compare every indexes at the right of the index (i) to find the smallest value among the values that are smaller than arr[i] if it exists.
Then, we swap the element at the smallest_index position and i.
Thus, if there is no smaller element among the unsorted part, we will continue to next step without doing anything.
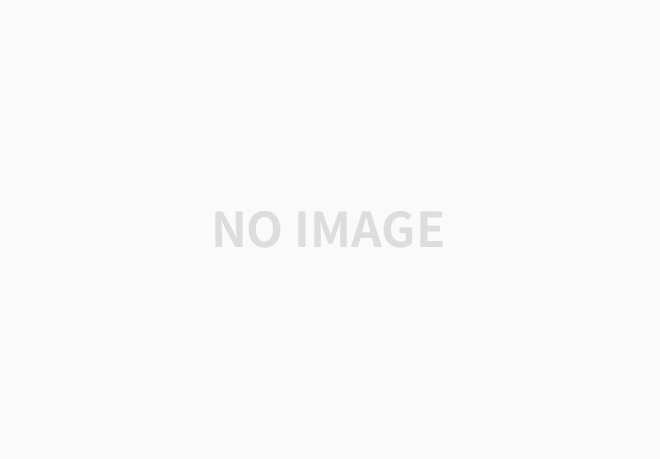
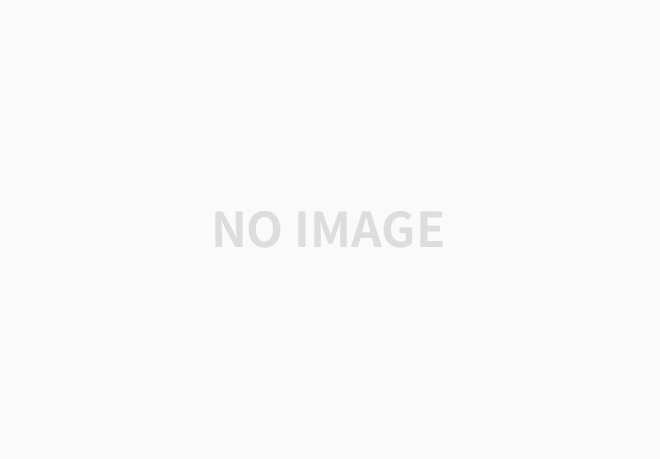
The implemented function in Python would be like this.
def selection_sort(arr: list[int]) -> None:
for i in range(len(arr)):
index_smallest = i
for j in range(i + 1, len(arr)):
if arr[index_smallest] > arr[j]: index_smallest = j
arr[i], arr[index_smallest] = arr[index_smallest], arr[i]
We could start from the right, and sort from the right of the array.
We could also sort in descending order by looking for the largest index instead of the smallest.
Time Complexity of Selection Sort
As you can guess from the fact that selection sort requires a looped traverse, it would have a comparatively large runtime complexity.
The selection sort algorithm's runtime complexity is in fact, O(N²).
If the array has N elements, the outer loop (i) with execute for N - 1 times.
For each loop, there will be an average of N/2 execute of inner loop.
So it gives N(N - 1)/2 number of comparisons, or O(N²).
Interpreting the fact that T(N) = O(N²), 10 times of increase of the size of the data would result in about 100 times slower operation.
Similar to linear search, it has relatively simple algorithm but slower execution time compared to other sorting algorithms.
In the next post, we will look on to insertion sort algorithm.
'Data Science > Data Structure & Algorithm' 카테고리의 다른 글
[Sorting] Shell Sort (0) | 2025.03.17 |
---|---|
[Sorting] Insertion Sort (0) | 2025.03.17 |
[Algorithm] Big-O Notation | 빅 오 표기법 (0) | 2025.03.12 |
[Algorithm] Growth of Functions and Complexity (0) | 2025.03.12 |
[Algorithm] Constant Time Operation (0) | 2025.03.12 |